working_helen
[ Week 9 ] Processing User Input 본문
Lecture : Media Computation
Date : week 9, 2024/04/29
Topic : Processing User Input
1. <form> 태그
2. 다양한 user-interface 태그
3. User Feedback Form 실습
1. <form> 태그
- 입력된 여러 데이터를 한번에 서버로 전송해주는 기능을 수행
- form 태그의 다양한 attribute
- action : form이 전송할 데이터를 받을 서버(url, 스트립트 파일) 지정
- accept-charset : form 전송시 사용될 문자 인코딩 지정
- method : 폼을 서버에 전송할 때 사용할 http 메소드를 지정
- 다양한 user-interaface 태그들(input, button, select 등)과 함께 사용됨
- 'required' : form의 하위 태그들에 'required' 속성을 적용하면 해당 태그에 데이터가 반드시 입력되어야 form이 데이터를 전송할 수 있도록 설정 가능
<form action="url 주소" method="get" accept-charset="utf-8">
<label for="name">Enter your name: </label>
<input type="text" name="name" id="name" required />
<label for="email">Enter your email: </label>
<input type="email" name="email" id="email" required />
</form>
2. 다양한 user-interface 태그
1) <input> 태그의 다양한 type
- input type="text" : 한 줄 텍스트 입력창
// minlength, maxlengthv : 입력 string의 최소, 최대 길이 지정
<input type="text" minlength="4" maxlength="8" size="10" required/>
- input type="email" : 이메일 주소 입력창
// pattern : valid 하다고 인식되는 email 형식
<input type="email" pattern=".@website.com"/>
- input type="number" : 숫자만 입력할 수 있도록 한정
// min, max : 입력 가능한 최소, 최대 숫자
<input type="number" min="10" max="100" />
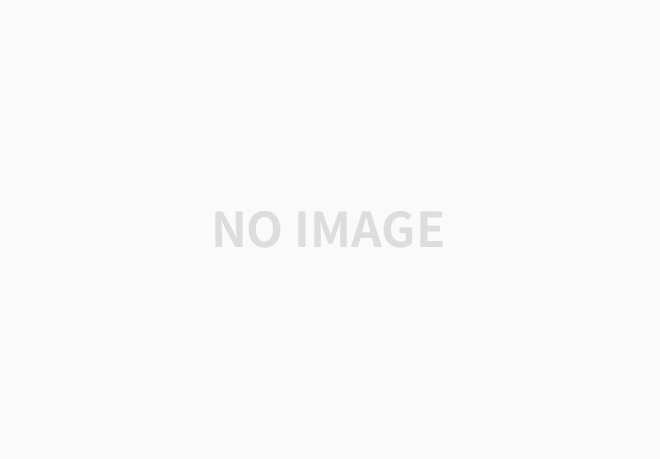
- input type="date" : 연, 월, 일 선택창
// value : 사용자 입력 전 기본적으로 보여지는 날짜
// min, max : 최소, 최대 선택 가능 날짜
<input type="date" value="2024-04-01" min="2024-01-01" max="2024-12-31"/>
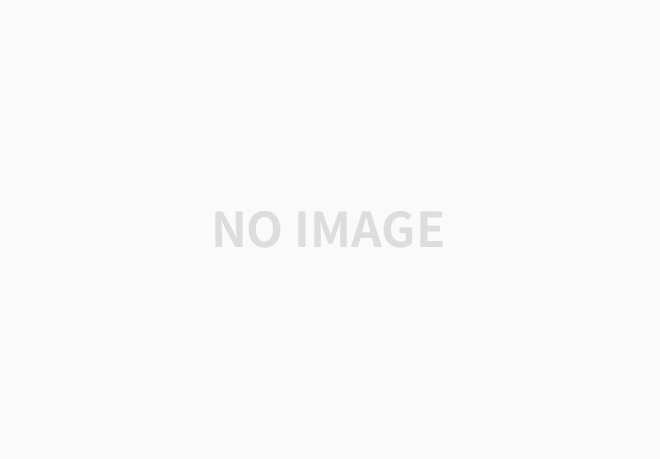
- input type="radio" : 선택 그룹의 옵션 버튼, 그룹 내 하나만 선택 가능
// name : 같은 그룹에 속하는 옵션들이 공유하는 그룹 이름
// value : 각 옵션의 고유한 값
// checked : 사용자 입력 전 기본적으로 선택되어 있는 옵션
<input type="radio" id="apple" name="fruit" value="apple" checked />
<label for="apple">apple</label>
<input type="radio" id="orange" name="fruit" value="orange" />
<label for="orange">orange</label>
<input type="radio" id="banana" name="fruit" value="banana" />
<label for="banana">banana</label>

- input type="checkbox" : 선택 상자 버튼, radio와 달리 그룹화 되지 않고 사용될 수 있으며 여러개를 동시에 선택 가능
// name : 각 선택 상자의 고유한 값
// radio와 달리 그룹를 지정하지 않으며, 여러개를 동시 선택 가능
<input type="checkbox" id="apple" name="apple" checked />
<label for="apple">apple</label>
<input type="checkbox" id="banana" name="banana" />
<label for="banana">banana</label>
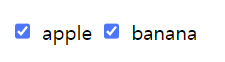
- input type="submit" : 버튼의 일종으로 click 이벤트가 발생하면 form의 데이터를 서버로 전송
※ onsubmit : form 내부에 <input type="submit">가 존재하는 경우, 해당 태그의 이벤트가 발생할 때 수행할 함수 동작을 지정
// value : submit 버튼 위에 쓰여질 문구
<input type="submit" value="Send Data" />
<form onsubmit="submit 버튼 click시 수행할 함수">
form 내 각종 user-interface 태그들
<input type="submit" value="Submit"/>
</form>
2) <select>과 <option>
<label for="fruits">Choose a fruit:</label>
<select name="fruits" id="fruits">
// value를 빈 값으로 지정하면 사용자 선택 전 기본적인 선택 사항을 지정
<option value="">--Please choose one fruit--</option>
// value : 각 옵션의 고유한 값 지정
<option value="apple">apple</option>
<option value="orange">orange</option>
<option value="banana">banana</option>
</select>
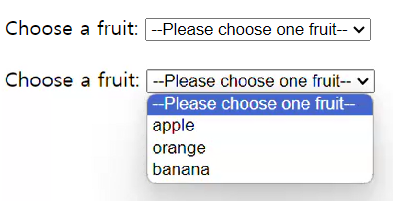
3. User Feedback Form 실습
- form의 하위 태그에 'required' 속성을 추가
→ 데이터가 입력되지 않으면 form이 데이터를 서버에 넘길 수 없도록 설정
- submit 버튼에 click 이벤트 발생
→ form은 action에서 지정한 url에 데이터를 전송 + 동시에 onsubmit에서 지정한 함수를 수행
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script>
// rating 값을 확인하고 문구를 출력해주는 함수
function submitForm() {
let rating = document.querySelector("#rating").value
if(rating < 5){
alert("Your rating is " + rating + ". Thank you!")
} else {
alert("Your rating is " + rating + ". Thank you for perfect score!")
}
}
</script>
</head>
<body>
<h1>User Feedback Form</h1>
<!-- sumbit 버튼이 눌리면 전체 form에 대하여 함수 실행 -->
<form onsubmit="submitForm()">
<label for="name">Your name:</label><br />
<input type="text" id="name" name="id" required><br />
<label for="email">Your email:</label><br />
<input type="email" id="email" name="email" size="30" required/><br />
<label for="postcode">Your postcode:</label><br />
<input type="number" id="postcode" name="postcode" min="1000" max="4000" required><br />
<label for="product">Your product purchased:</label><br />
<select id="product" name="product" required>
<option value="">Select a product</option>
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="orange">Orange</option>
<option value="peach">Peach</option>
<option value="lemon">Lemon</option>
</select><br />
<label for="date">Your date purchased:</label><br />
<input type="date" id="date" name="date" required><br />
<label for="comment">How would you describe your experience?</label><br />
<textarea id="comment" name="comment" rows="5" cols="40" required></textarea><br />
<label for="rating">Your 1-5 rating:</label><br />
<input type="number" id="rating" name="rating" min="1" max="5" required><br />
<input type="submit" value="Submit"/><br />
</form>
</body>
</html>
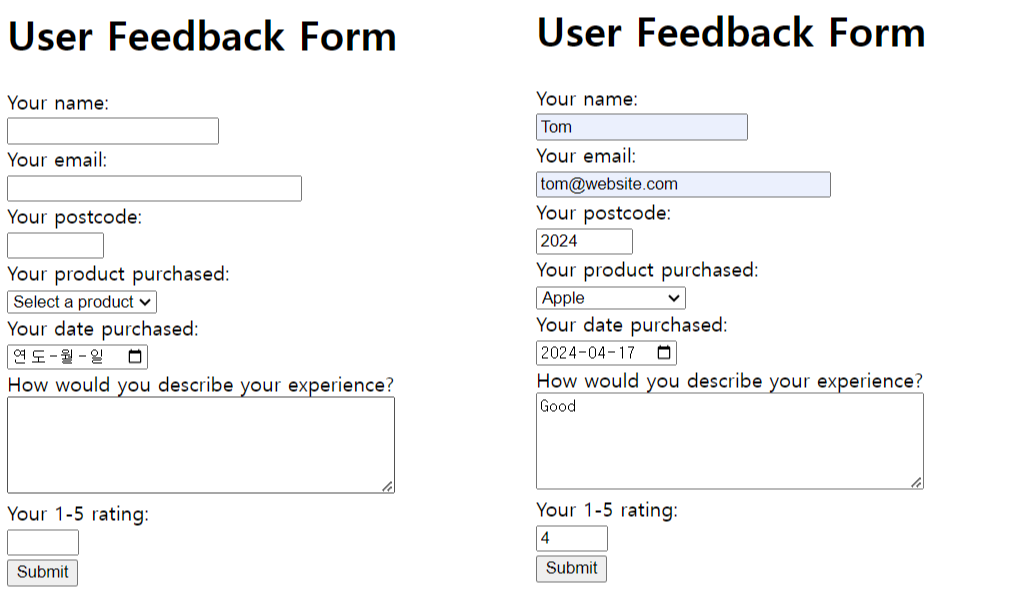
reference
https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input
https://developer.mozilla.org/en-US/docs/Web/HTML/Element/select
https://www.nextree.co.kr/p8428/
'교내 수업 > Media Computation' 카테고리의 다른 글
[ Week 7 ] JS Arrays and Structured Data (0) | 2024.04.19 |
---|---|
[ Week 4 ] Java Programming Essentials (0) | 2024.03.17 |
[ Week 3 ] Style with CSS (0) | 2024.03.08 |
[ Week 2 ] Structure with HTML (0) | 2024.03.05 |